How to display a grid of images
Say you want to display 12 images in a grid view. How would you do it without skewing the images and making sure they each fit their square?
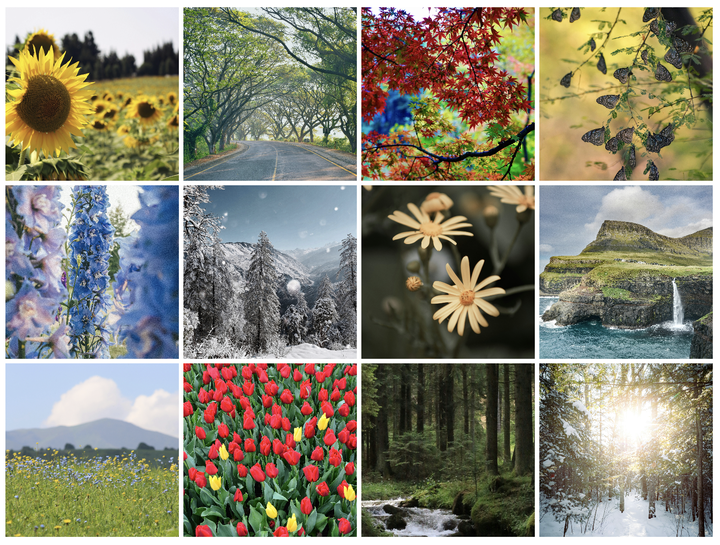
The easiest way is using CSS Grid.
Let's go over it below, first as plain CSS and then using Tailwind.
Here is the HTML markup we will be using:
<div class="image-gallery">
<img src="https://images.unsplash.com/photo-1626808642875-0aa545482dfb" />
<img src="https://images.unsplash.com/photo-1613479267503-eafa8b242987" />
<img src="https://images.unsplash.com/photo-1590639815345-f30dd48aba1b" />
<img src="https://images.unsplash.com/photo-1615300236079-4bdb43bd9a9a" />
<img src="https://images.unsplash.com/photo-1625278390415-f07c7fbd30b3" />
<img src="https://images.unsplash.com/photo-1622607672369-d8a81e658318" />
<img src="https://images.unsplash.com/photo-1616501998639-04447c1dd713" />
<img src="https://images.unsplash.com/photo-1493962621260-74cc5a3baf89" />
<img src="https://images.unsplash.com/photo-1617985562309-2aa7781f1608" />
<img src="https://images.unsplash.com/photo-1518813623778-bf4eaa951e6a" />
<img src="https://images.unsplash.com/photo-1610194435904-cdd826cc99b8" />
<img src="https://images.unsplash.com/photo-1611189986247-9c5c009d4003" />
</div>
Before we apply any styling, this is how the images look like:
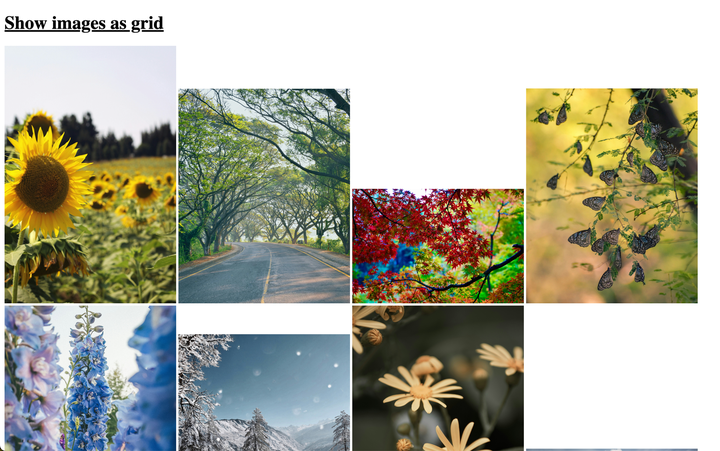
Using plain CSS
Use display grid to show 4 columns
To display the image gallery as a grid, we can use the display:grid
property and show 4 equal width columns:
.image-gallery {
display: grid;
grid-template-columns: repeat(4, 1fr);
}
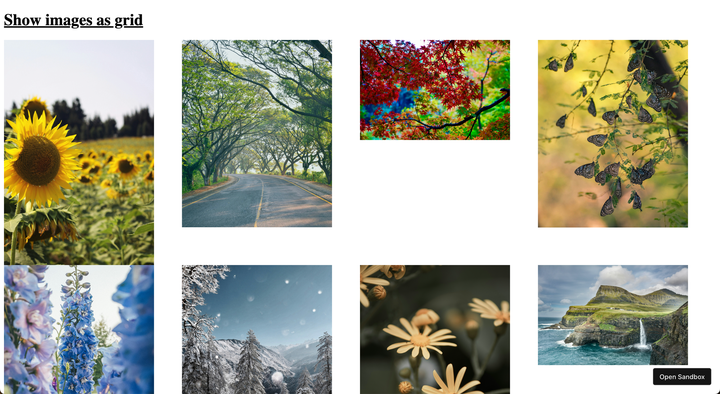
Show images as full width to fill the page
We can use set the width and height of the images to 100%, so the gallery looks more like a grid:
.image-gallery img {
width: 100%;
height: 100%;
}
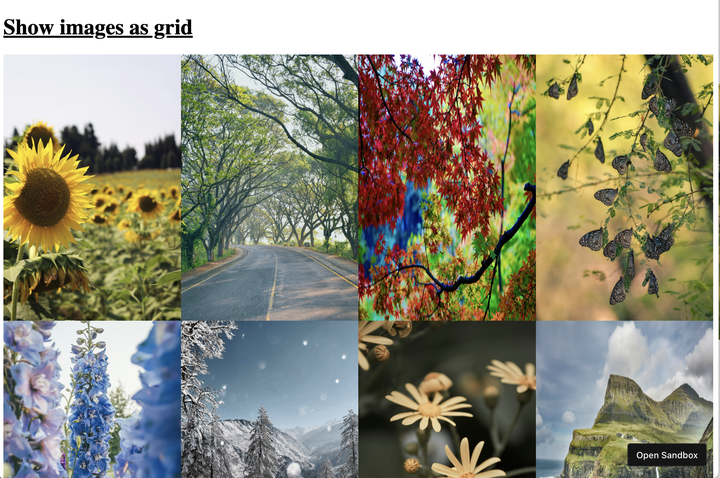
Fix skewing using object-fit
The CSS object-fit
property allows us to specify how the image should fill the available space - by default this is stretch
, which is why the images were skewed above. We can set it to cover
to fix this:
.image-gallery img {
...
object-fit: cover;
}
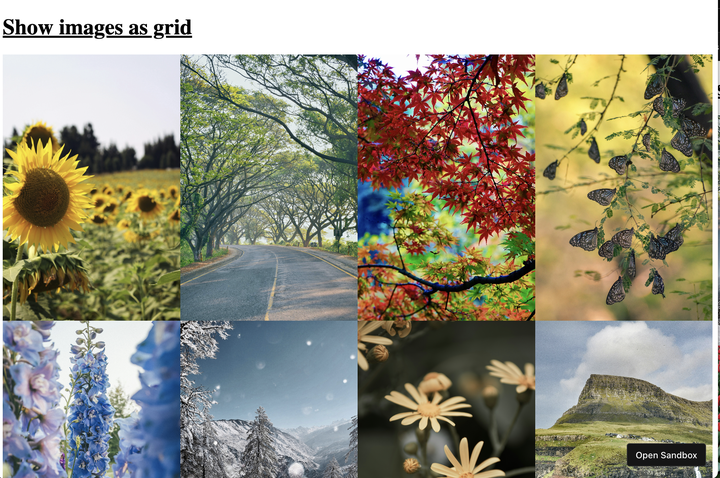
Add grid gap for spacing between images
It would be nice to have some spacing between the images - CSS grid has a built in property for thus - gap
:
.image-gallery {
...
gap: 5px;
}
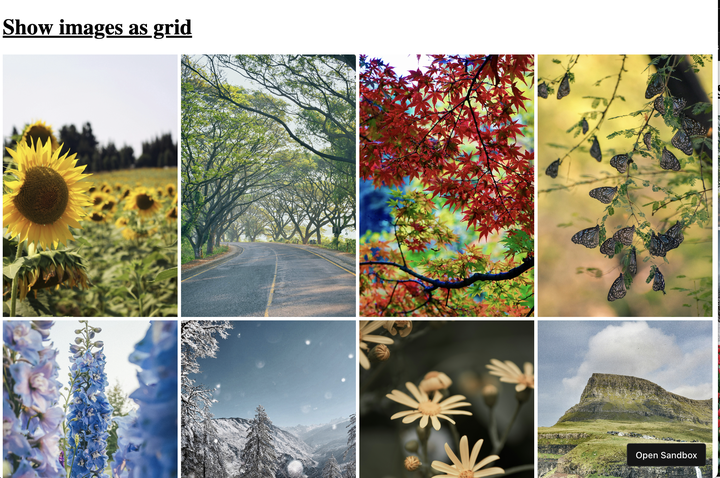
Show as squares using aspect ratio
Our gallery is almost done! But it would be nice to have the images shown as squares, instead of portrait as now. We can achieve that using the aspect-ratio
property:
.image-gallery img {
...
aspect-ratio: 1;
}
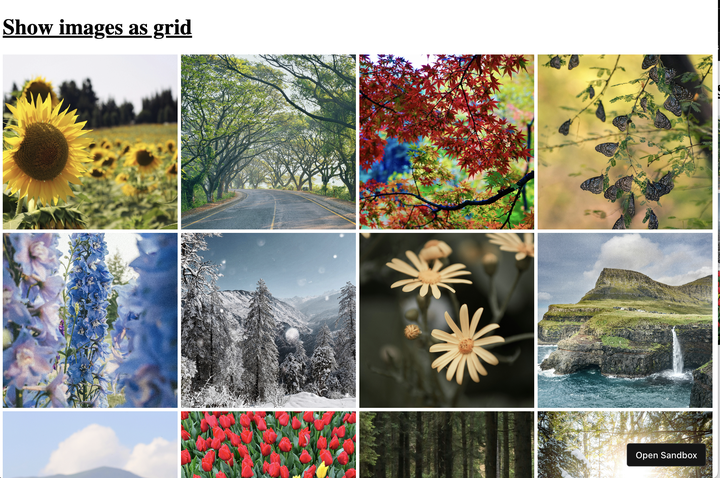
Keep images together even on wide screens
If you are viewing this on a wide screen, you'll probably notice the images are spaced out on the x axis. To fix this, we can add a width
of fit-content
to the image gallery, so it does not expand to fit the whole screen:
.image-gallery {
width: fit-content;
}
Done!
And that's it! Here is the full CSS:
.image-gallery {
display: grid;
grid-template-columns: repeat(4, 1fr);
gap: 5px;
width: fit-content;
}
.image-gallery img {
width: 100%;
height: 100%;
object-fit: cover;
aspect-ratio: 1;
}
Using Tailwind CSS
How would you implement this with Tailwind?
<div class="grid grid-cols-4 gap-1">
<img
class="w-full h-full object-cover aspect-square"
src="https://images.unsplash.com/photo-1626808642875-0aa545482dfb"
/>
...
</div>
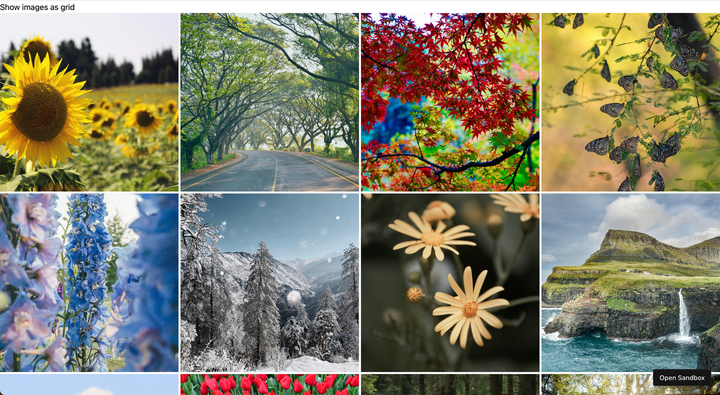
Practice this yourself!
Did you enjoy this walkthrough? Put your understanding to the test by building a memory game! The images will be displayed using exactly this technique.
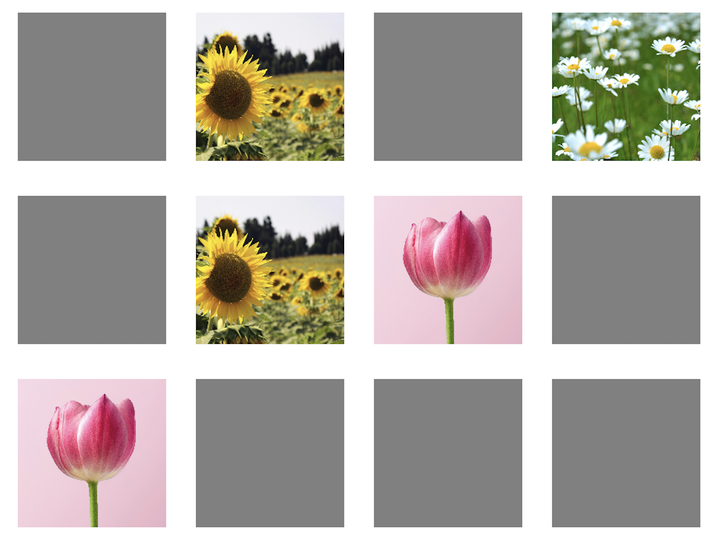
Member discussion