Build a custom useFetch hook
Build a custom useFetch
hook that encapsulates the logic for handling the loading and error states when fetching data:
const { data, isLoading, error } = useFetch(SOME_URL, MAYBE_OPTIONS);
The code should
- return the response from the server
- handle error and loading states
- support custom headers through an options parameter
- support all HTTP methods - e.g. both GET and POST requests
Use Typescript to build your solution.
Here is a sample PokemonList
component that uses the hook:
import { useFetch } from "./use-fetch";
type Pokemon = {
name: string;
};
const PokemonList = () => {
const { data, isLoading, error } = useFetch<{ results: Pokemon[] }>(
`https://pokeapi.co/api/v2/pokemon?${new URLSearchParams({
limit: "10",
offset: "0",
})}`
);
const pokemons = data?.results || [];
if (isLoading) {
return <p>Loading ...</p>;
}
if (error) {
return <p>{error}</p>;
}
return (
<ol>
{pokemons.map((pokemon) => (
<li key={pokemon.name}>{pokemon.name}</li>
))}
</ol>
);
};
export default PokemonList;
To help you get started, the starter repo includes failing unit tests for both the useFetch
hook and the PokemonList
component.
GitHub - reactpractice-dev/use-fetch-hook
Contribute to reactpractice-dev/use-fetch-hook development by creating an account on GitHub.
💡
Ready to check your work?
Become a member and get access to the official solution and the comments section for feedback and discussion.
Become a member and get access to the official solution and the comments section for feedback and discussion.
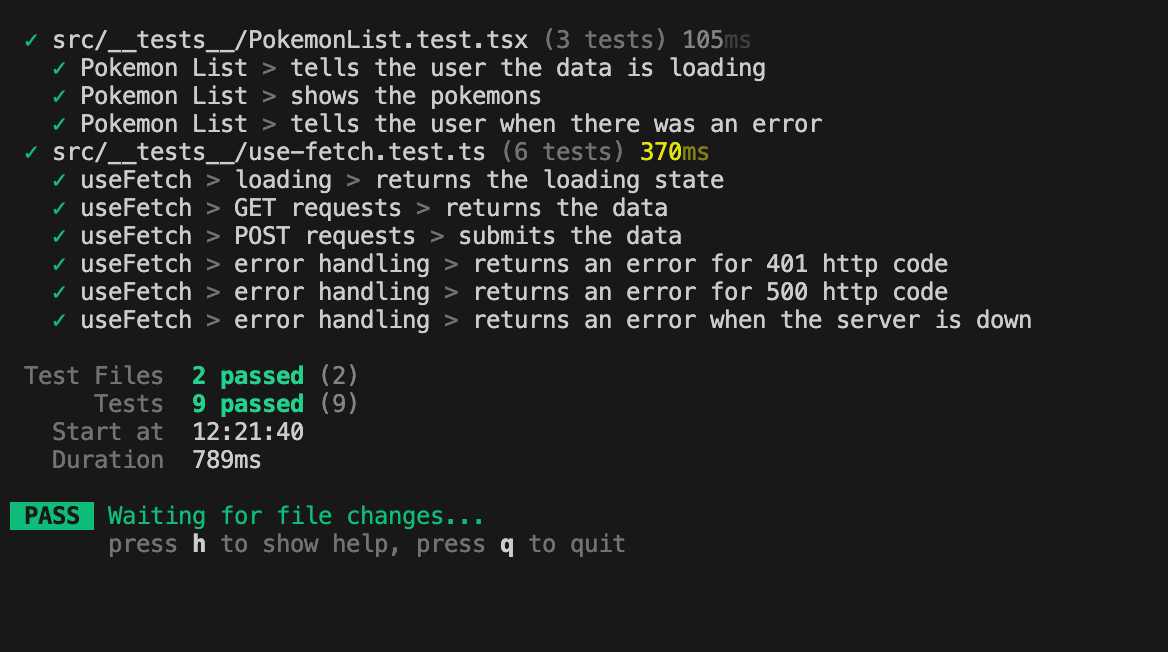
Member discussion