Build a notes app with React Query and json server - starting from failing unit tests!
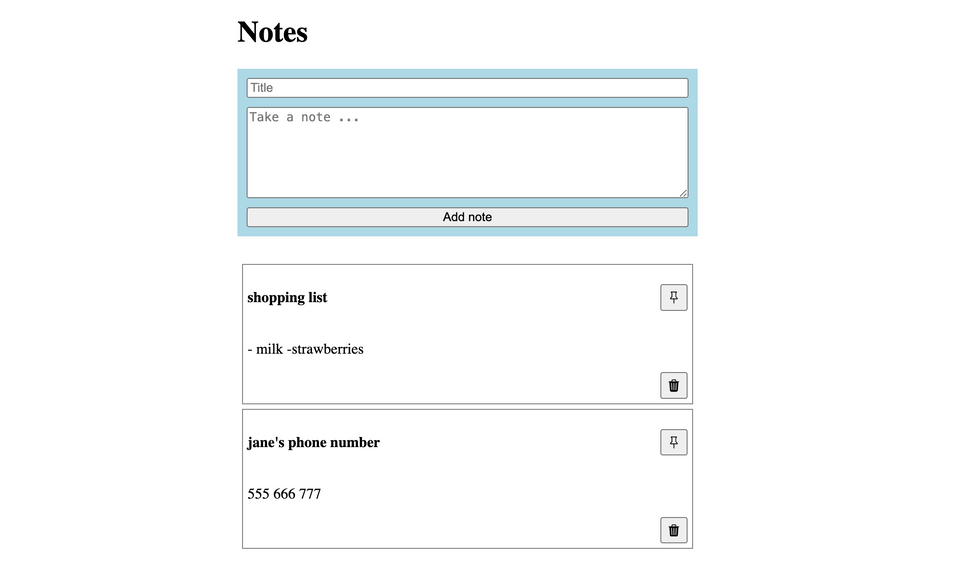
Build a Google Keep inspired Notes app using React Query for fetching the data and json-server
as the backend API.
- Users can add notes with a title and text content
- Notes are shown on top of each other, with the latest one on top
- Users can "pin" notes; pinned notes are always shown on top
- Users can delete notes by clicking a trash icon next to the note
- Users can edit notes by clicking them
To allow for a smooth user experience, take note of these points as well:
- Show a loading indicator when saving a note is in progress (add/edit/delete) - by disabling the form button and changing its label (e.g. "Delete note" -> "Deleting note")
- Show "toast" messages for success and error
The boilerplate already has the backend server setup and a full test suite to make sure all the requirements are covered.
Clone the repo and see if you can get the tests to pass!
Notes:
- Styling doesn't matter for the purpose of this exercise, so it's ok to keep things very barebones.
- Recommended libraries:
axios
for data fetching,react-icons
for the icons,react-hot-toast
for the "toast" messages - When sorting the notes to show the latest one on top, assume the backend always returns them in a chronological order (since we don't have a "created date" to sort by).
- Use Chrome's
Network tab > throtling
to delay requests, so you can see the loading messages in development. - If you want to learn more about using
json-server
as a backend API, check out this article that goes over it in detail.
Implementation notes
- for the note form - add
aria-label
to the form fields -Title
andContent
respectively - for the notes list - use an
ul
for the notes (i.e. each note should be wrapped in anli
element); use a heading (e.g.h3
) for the note title
The unit tests expect a certain component structure, so it helps if you follow it. The boilerplate already contains the empty components.
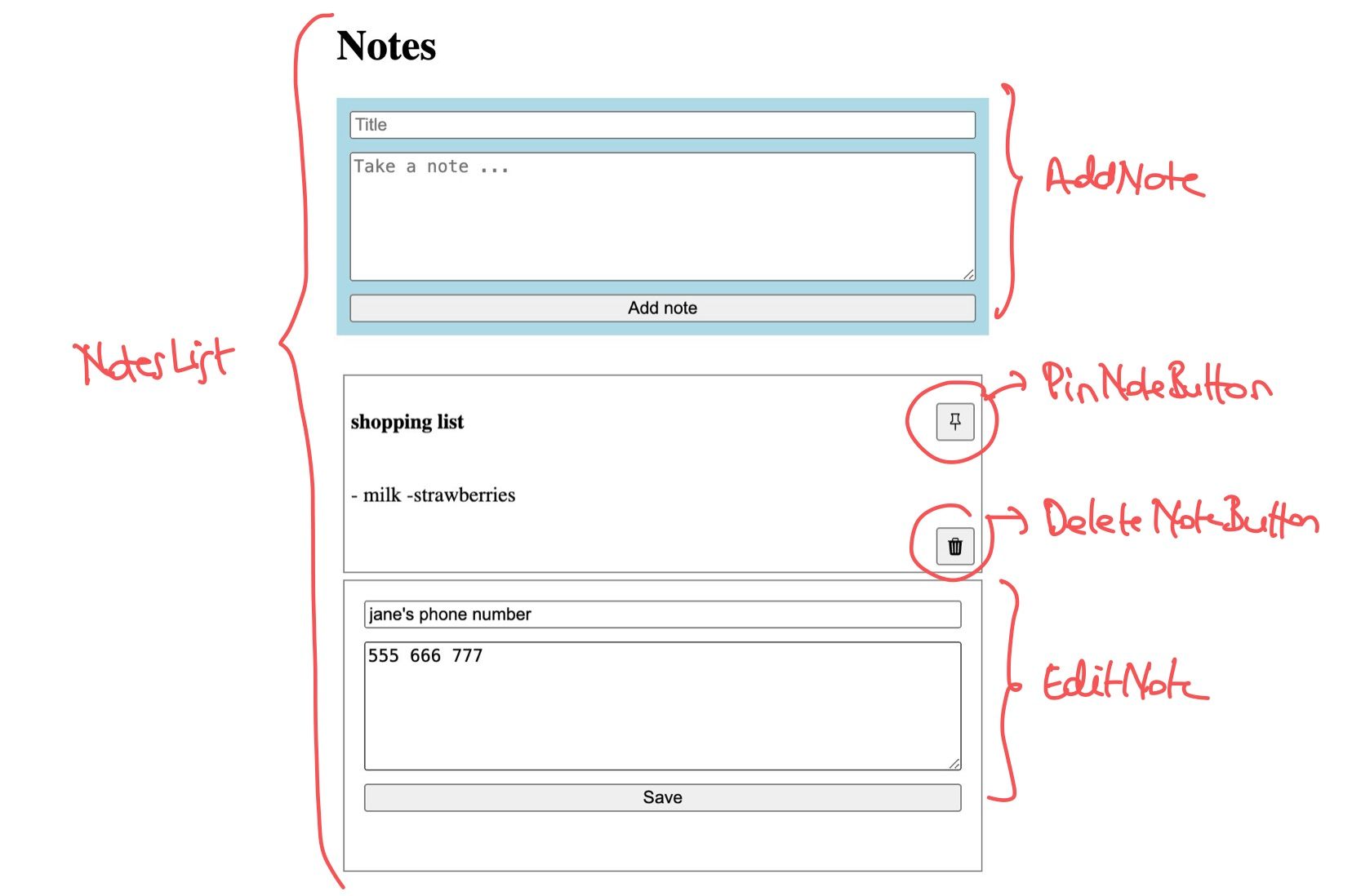
Good luck!
Become a member and get access to the official solution and the comments section for feedback and discussion.
Member discussion